10 December 2012
Gear Rotation Animation Python Script
This script takes a pre-drawn group of gear outlines (generated using the CamBam gear generator), then
animates them using a series of relationship rules within the script.
This script requires CamBam 0.9.8N or later.
This script demonstrates:
- Importing a helper Python module.
- Defining a Python class.
- Animating objects by changing their transformation property.
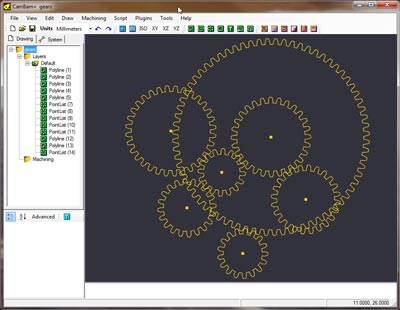
Source
Download
This download also contains the example CamBam drawing required by this script.
gear-animate-source.zip (89 KB)
gear_animate.py
import gears
from gears import *
xgear = [ geardef(1,11,16,2),
geardef(2,7,20,2),
geardef(3,8,24,2),
geardef(4,9,28,2),
geardef(5,10,32,2),
geardef(12,9,72,2),
geardef(13,14,17.0,2) ]
xgear[1].link( xgear[0] )
xgear[3].link( xgear[0] )
xgear[4].link( xgear[0] )
xgear[2].link( xgear[3] )
xgear[5].speed = xgear[3].speed
xgear[6].link( xgear[5] )
th = Math.PI/180.0
for dd in range (1,360):
for xg in xgear:
xg.rotate(th)
view.RefreshView()
app.Sleep(10)
for xg in xgear:
xg.reset()
gears.py
from CamBam.Geom import *
class geardef:
def __init__(self,_id,_center,_teeth,_pitch):
self.id = _id
self.teeth = _teeth
self.pitch = _pitch
self.d = _teeth / _pitch
self.ent = doc.FindPrimitive(_id)
cp = doc.FindPrimitive(_center)
self.c = cp.Points[0]
self.speed=1.0
def link(self,other):
self.speed = -other.speed * other.d / self.d
def rotate(self,theta):
self.ent.Transform.Translate(-self.c.X,-self.c.Y,0)
self.ent.Transform.RotZ(theta*self.speed)
self.ent.Transform.Translate(self.c)
def reset(self):
self.ent.Transform = Matrix4x4F.Identity